create a 3D model in Python
To create a 3D model in Python, you can use libraries such as
matplotlib
for basic 3D plotting or more specialized libraries like Mayavi
or PyOpenGL
for more advanced 3D graphics. Here's a simple example using matplotlib
to create a 3D plot: 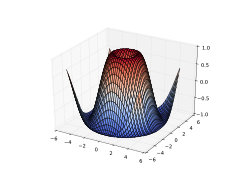
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
# Create a figure and a 3D axis
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# Generate data
theta = np.linspace(-4 * np.pi, 4 * np.pi, 100)
z = np.linspace(-2, 2, 100)
r = z**2 + 1
x = r * np.sin(theta)
y = r * np.cos(theta)
# Plot 3D curve
ax.plot(x, y, z, label='3D Spiral')
# Add labels and a legend
ax.set_xlabel('X axis')
ax.set_ylabel('Y axis')
ax.set_zlabel('Z axis')
ax.legend()
# Show the plot
plt.show()
```
This example creates a simple 3D spiral using `matplotlib`. You can run this code in a Python environment that supports plotting, such as Jupyter notebooks or a Python script.
If you're looking to create more complex 3D models, especially for computer graphics or simulations, you might want to consider libraries like `Mayavi` or `PyOpenGL`. The specific approach would depend on the kind of 3D model you're trying to create (e.g., geometric shapes, CAD models, etc.).
No Comments have been Posted.